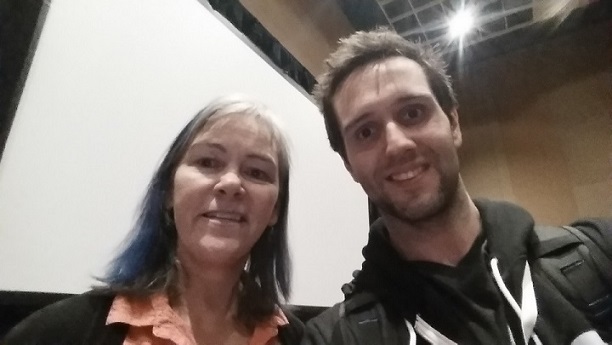
Well, that's a wrap! DEVintersection is over after a long week of sessions, technical difficulties, and an intense all-day workshop. I'm back in my hotel room at the MGM Grand, but we need to talk about everything that happened this week before I inevitably forget.
Docker all the things!
For starters, one thing is clear, Docker isn't going anywhere. Every speaker was leveraging Docker in some form in all of their sessions, even if the talk had nothing to do with it. Scott Hanselman gave one of the keynotes, Microsoft's open source journey, and he somehow managed to segway into demoing a raspberry pi cluster leveraging Kubernetes and Docker that hosted a .NET Core web application.
Coolness factor aside, it was actually pretty interesting to learn that .NET was actually "open sourced" a long time ago, the early 2000s I believe he said. Though it was just shared as a zip file and was intended for academia. The source code was sanitized as to not leak IP, and they refused to accept any contributions. Not that there were really any channels that would allow it. So in reality, it was more... "source opened".
So.. what exactly is DevOps?
After the keynote I attended a session called How DevOps Practices Can Make You a Better Developer and while the subject matter was pretty straightforward: defining what CI (continuous integration), CD (continuous delivery), etc were.. the biggest takeaway for me was Microsoft's definition of DevOps.
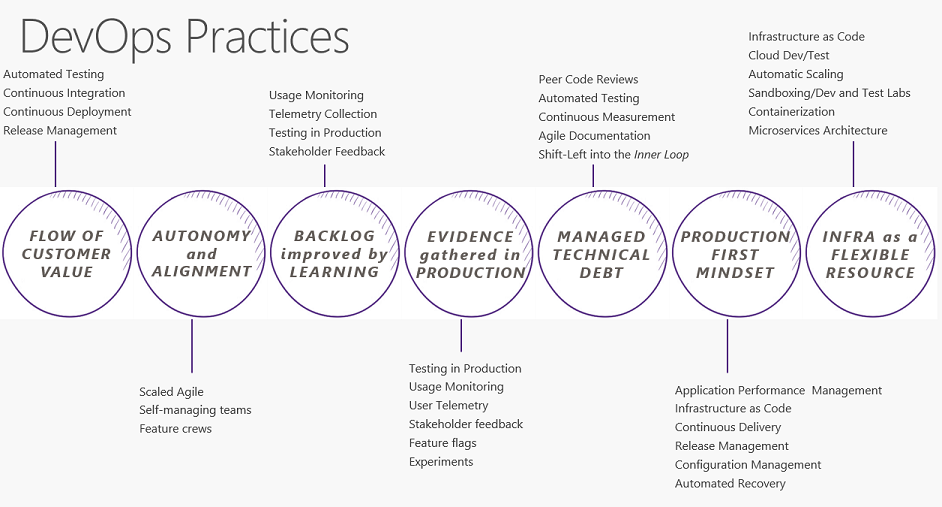
DevOps is the union of people, process, and products to enable continuous delivery of value to our end users. Donovan Brown, Microsoft DevOps PM
It made a lot of sense. DevOps is about so much more than just delivery. The above definition really highlights how so many companies can get DevOps "teams" wrong. It isn't a team! It's combining process and people together in order to deliver software to our users. That means that DevOps is not only delivery, but infrastructure, testing, monitoring, and so much more.
Another common theme? Microservices!
Yep, on top of containerization, microservices was another big discussion point of the conference. Which in all honesty, makes sense. The two go pretty well together.
I attended a few sessions relating to microservices, and though they were a little introductory, there were definitely some highlights I felt jotting down. One of the sessions I went to was hosted by Dan Whalin, a pretty big name in the JavaScript world, and he outlined a quick list of four questions to ask yourself before taking the plunge into microservices.
- Are multiple teams working on different business areas of the application?
- Is it hard for new team members to understand the application and contribute quickly?
- Are there frequently changing business rules?
- Can subsystems within the application be changed with a minimal amount of impact?
I believe there's a lot of value in taking a step back and questioning whether microservices are right for you and your project. Like everything else in our field, there are no silver bullets. Microservices are a good solution to an array of problems, but it's not one size fits all. The additional complexity, especially in infrastructure, may not be worth the investment if you don't answer "yes" to all of the above, or at least some.
To top off my microservices immersion, I also attended a session on implementing a microservices messaging architecture, specifically with Azure Event Hubs. Now, the session was cut pretty short. The speaker's presentation apparently relied heavily on the Internet for some demos, which.. did not get up and running for probably a good 30 minutes. They even attempted to use their cell phone to tether a connection. A little unfortunate, but I think they made their point.
Their main points? When using messaging and events, events are facts. They happened! And, you shouldn't necessarily immediately jump into breaking up your database architecture into consistent and eventually consistent. Start with everything going to the same destination (consistent store), and if/when you need to scale, you can take an eventually consistent approach.
I promise there were other session topics..
As of late, I've been reading and writing more functional code. It's a completely different way of thinking, and there are a lot of great benefits to doing so. I saw a session entitled Functional Techniques for C# and had to give it a go.
There were a lot of defining of terms, which I am completely OK with. I seem to always forget the definitions of words, so getting refreshers on currying and high order functions was a nice introduction to the talk.
The biggest takeaway I had from the session was the idea of Functional Refactoring. Now, we've all seen Imperative Refactoring. It's the type of refactoring that most of us are familiar with. It's where we pull out some code and shove it into a method, then call that method instead of the code.
Here's a quick refresher..
public void PrintResponse(int choice)
{
var output = string.Empty;
switch(choice)
{
case 1:
output = "Hello";
break;
case 2:
output = "Goodbye";
break;
}
Console.WriteLine(output);
}
Now in this .. case .. PrintResponse has two responsibilities: it has to figure out what the response is and then it has to print it. Applying some inside-out refactoring, we get the following solution:
public void PrintResponse(int choice)
{
var output = GetResponse(choice);
Console.WriteLine(input);
}
private string GetResponse(int choice)
{
var output = string.Empty;
switch(choice)
{
case 1:
output = "Hello";
break;
case 2:
output = "Goodbye";
break;
}
return output;
}
Now PrintResponse only has one job, to print the response. The job of getting the response has been abstracted away into a new private
method called GetResponse
Functional refactoring is a little different, again, it's outside-in rather than inside-out as I just showed. Here's a simple example:
public void Save()
{
try
{
context.Save();
}
catch(Exception ex)
{
Logger.Log(ex);
}
}
Applying functional refactoring..
public void Save()
{
Handling.TryWithLogging(() => context.Save());
}
public static void TryWithLogging(Func<DbContext> operation)
{
try
{
operation();
}
catch(Exception ex)
{
Logger.Log(ex);
}
}
So as you can hopefully see, we've taken the outside code, the using
block, and replaced it with a TryWithLogging
higher-order function. With this approach, you can wrap using
statements for calls to your database, wrap try
and catch
blocks to automatically log to the database on failure instead of copy pasting Logger.Log(ex);
at the end of every catch
.
Lastly an all day grind.. with C#!
I was excited about this workshop. I have honestly never done a workshop before at a conference, so I didn't really have any idea what to expect. All I knew was that it was going to be an all-day event, in a room, with the one and only Kathleen Dollard.
There was so much information in this workshop, it might even need to be a blog post all by itself, but I wanted to highlight some of the key points that I felt stood out and that I resonate with.
The first part of the workshop really focused on teams and learning. Improving yourself and others. She spoke to that research has shown that within a team, the who didn't actually matter, that is, the team's diversity. But rather, teams performed better if everyone spoke around the same amount and that there was psychological safety (no fear of belittlement during a review or just for speaking up). It's interesting to think about anyway, as I feel most of us are conditioned to believe that diversity plays a big role in the success of a given team.
She also spoke about cognitive dissonance. Which essentially states that you will take new input and associate it with your current perception. Seems obvious when it's put like that, but it makes a lot of sense and really shows why things such as code reviews are so important. We really need to be challenging what we know, day to day, so we can take that new information and apply it correctly.
For example, I once had this preconceived notion a long time ago that several meant seven in mathematics. This perception caused me to get stuck on a particular mathematics problem for quite some time because I just couldn't reason about how the problem could even be solved. The question didn't make any sense in the context of several being seven. The world was much more clear to me when I was corrected. This is why code reviews are so beneficial. If our perception is wrong, and we stay in our bubbles, we will continue to be wrong.
Now, all of that really has little to do with C#, but I found it immensely helpful as a software professional. The tips and tricks were great but mostly focused on functional techniques that I discussed previously, just in greater detail.
All good things must come to an end...
And with that, the conference was over. I got to spend a good 30 minutes talking with Kathleen after everyone else had left the workshop room about her new position at Microsoft and what they were up to. Most others had to catch flights or had other arrangements. I for some reason thought the red eye was a good idea and wouldn't be leaving until 10:00 that night..
Until the next conference!